Updating CRM custom fields when creating a new booking
Update a customer's CRM custom fields when creating a new booking via the Appointedd API.
You can set up your Appointedd organisation's CRM to contain custom fields that can be recorded on a customer's record. To use CRM custom fields you'll first need to set them up in your Appointedd organisation which you can do by following our support guide How to ask your customers for additional personal details which covers how to do this.
Once you've have these custom CRM fields set up you can now include them in your payload when you make a request to create a booking via the Appointedd API. Here's an example request that updates two different custom fields on a customer whilst creating a booking for them at the same time (using a reservation slot that you previously created, see the previous guide on Creating a new booking for how to do this):
const axios = require("axios").default;
const options = {
method: 'POST',
url: 'https://api.appointedd.com/v1/bookings',
headers: {Accept: 'application/json', 'Content-Type': 'application/json'},
data: {
data: {
customers: [
{
customer: {
profile: {firstname: 'Ada', lastname: 'Lovelace', email: '[email protected]'},
custom_fields: [
{id: 'membership_id', value: 'ada-0123456'},
{id: 'birthday', value: '1994-07-15T00:00:00.000Z'}
]
},
}
]
},
slot_id: '<SLOT_ID>'
}
};
axios.request(options).then(function (response) {
console.log(response.data);
}).catch(function (error) {
console.error(error);
});
In this example we are setting two custom field values for our customer that have ben configured in our CRM. It's important that the id
property of the custom field matches the custom field that you are setting in your organisation. You can find this in the Custom Fields table in your organisation's CRM settings page. The id
to use for a custom field is listed under that field's Field ID column.
Here's an example of what that looks for the above example custom fields.
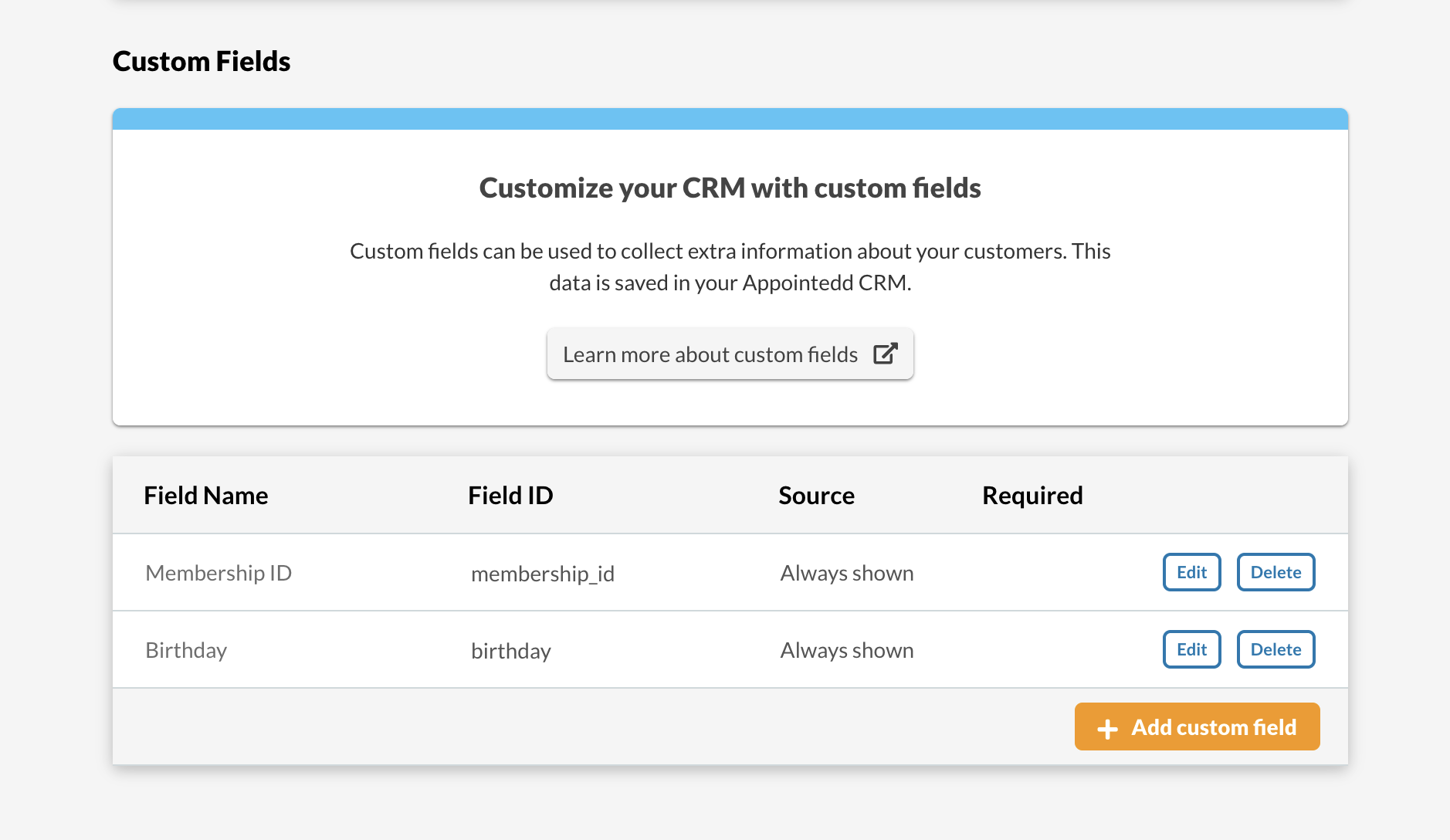
It is important that if you created a custom field set to the "date"
type, which in our example the "birthday"
custom field uses this type, then the value must be a valid ISO8601 string in order for it to be saved and rendered correctly in Appointedd.
Updated over 3 years ago